Making Decisions in Visual Basic Code What You’ll

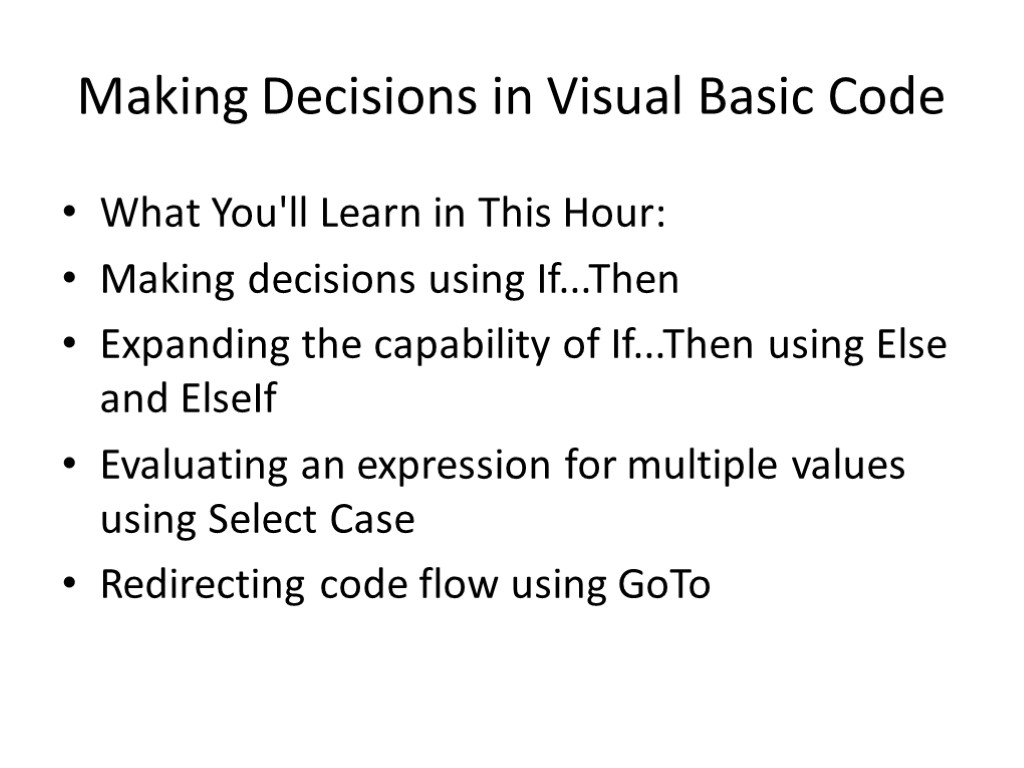
Making Decisions in Visual Basic Code What You'll Learn in This Hour: Making decisions using If...Then Expanding the capability of If...Then using Else and ElseIf Evaluating an expression for multiple values using Select Case Redirecting code flow using GoTo
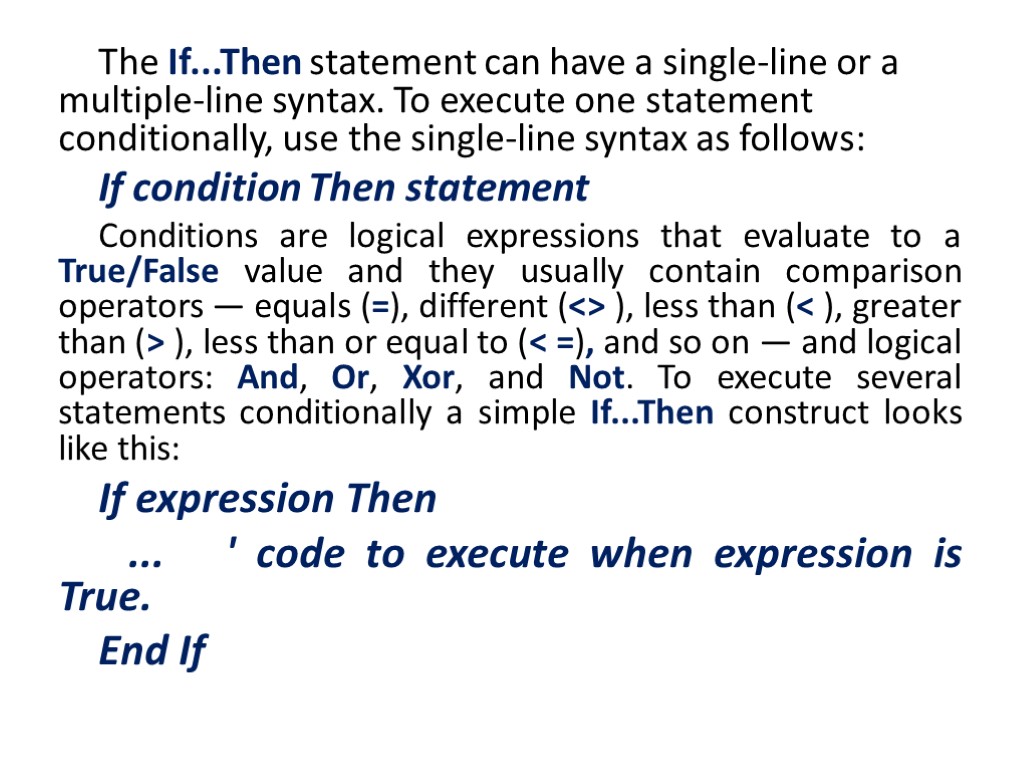
The If...Then statement can have a single-line or a multiple-line syntax. To execute one statement conditionally, use the single-line syntax as follows: If condition Then statement Conditions are logical expressions that evaluate to a True/False value and they usually contain comparison operators — equals (=), different (<> ), less than (< ), greater than (> ), less than or equal to (< =), and so on — and logical operators: And, Or, Xor, and Not. To execute several statements conditionally a simple If...Then construct looks like this: If expression Then ... ' code to execute when expression is True. End If
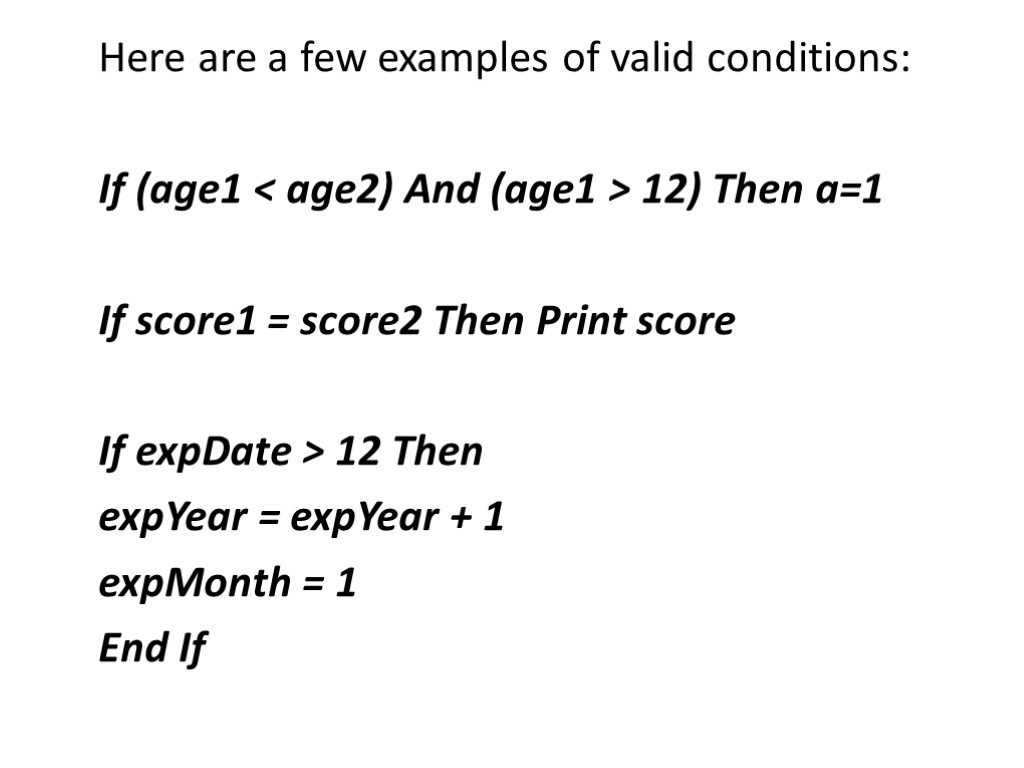
Here are a few examples of valid conditions: If (age1 < age2) And (age1 > 12) Then a=1 If score1 = score2 Then Print score If expDate > 12 Then expYear = expYear + 1 expMonth = 1 End If
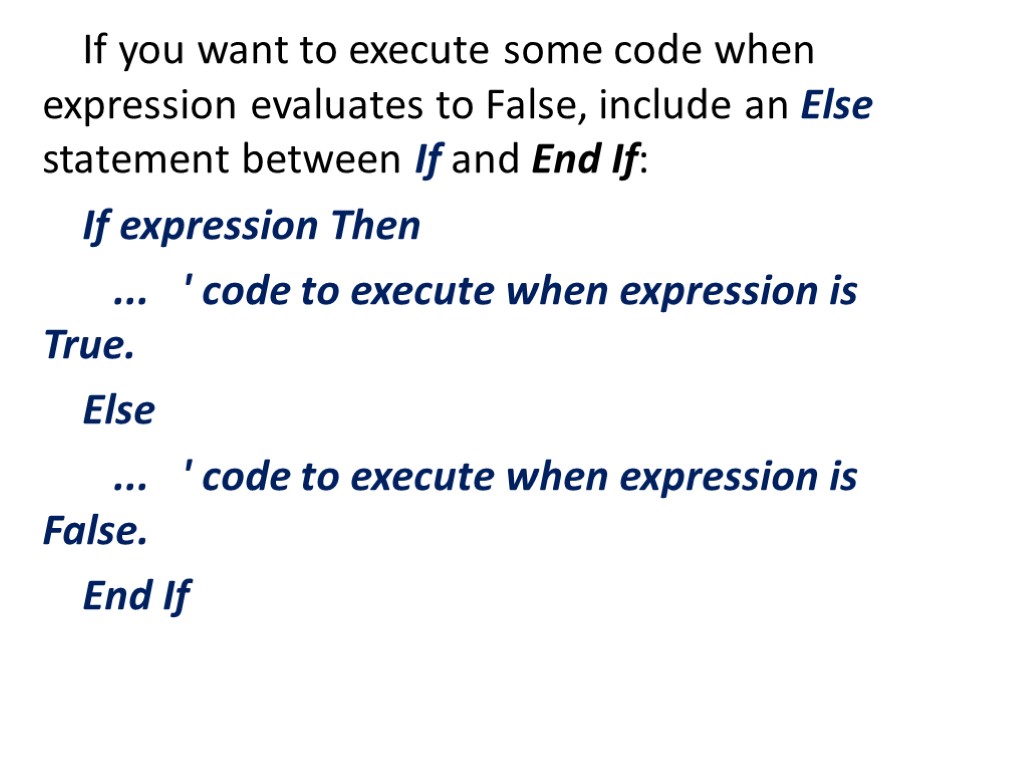
If you want to execute some code when expression evaluates to False, include an Else statement between If and End If: If expression Then ... ' code to execute when expression is True. Else ... ' code to execute when expression is False. End If
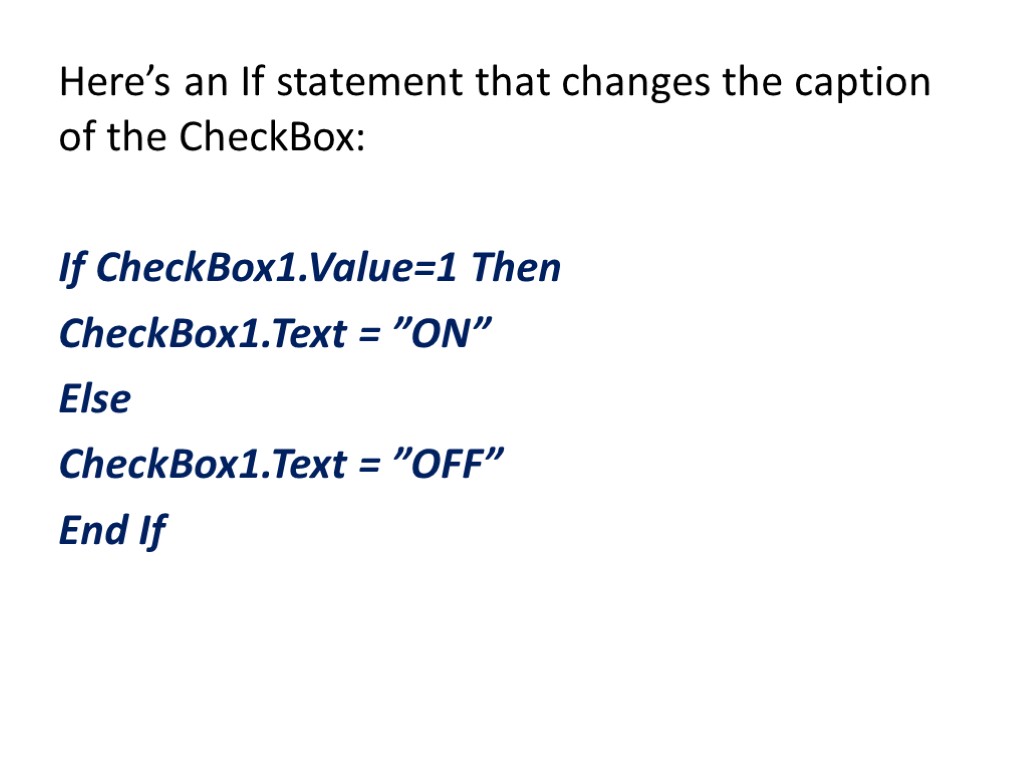
Here’s an If statement that changes the caption of the CheckBox: If CheckBox1.Value=1 Then CheckBox1.Text = ”ON” Else CheckBox1.Text = ”OFF” End If
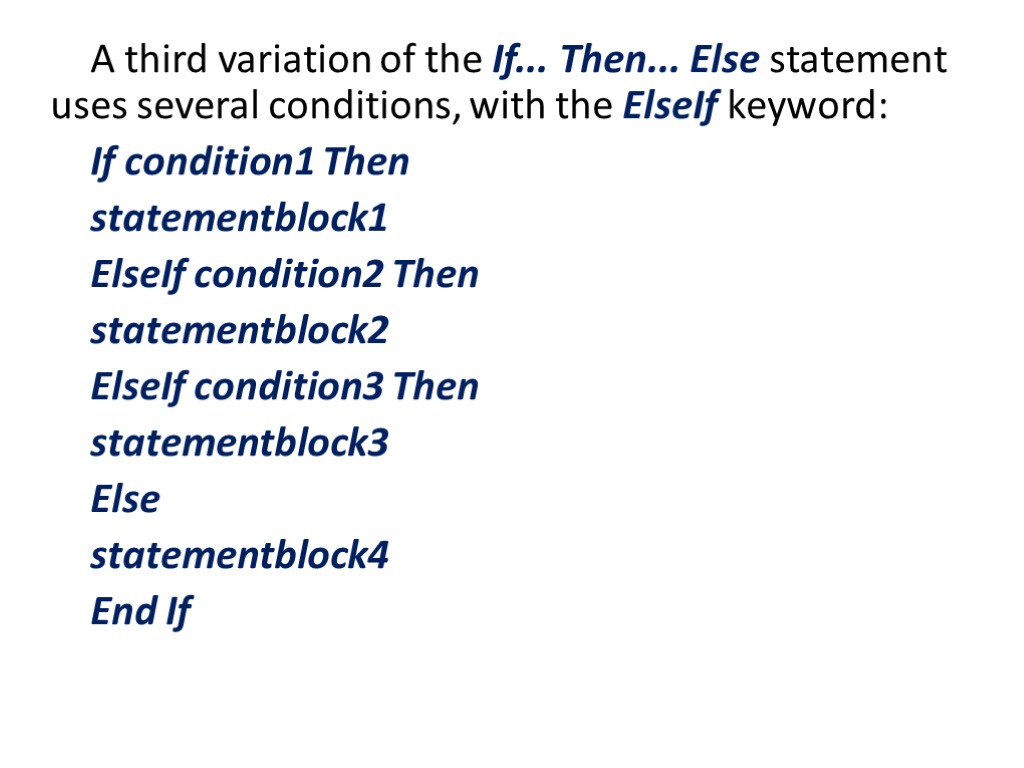
A third variation of the If... Then... Else statement uses several conditions, with the ElseIf keyword: If condition1 Then statementblock1 ElseIf condition2 Then statementblock2 ElseIf condition3 Then statementblock3 Else statementblock4 End If
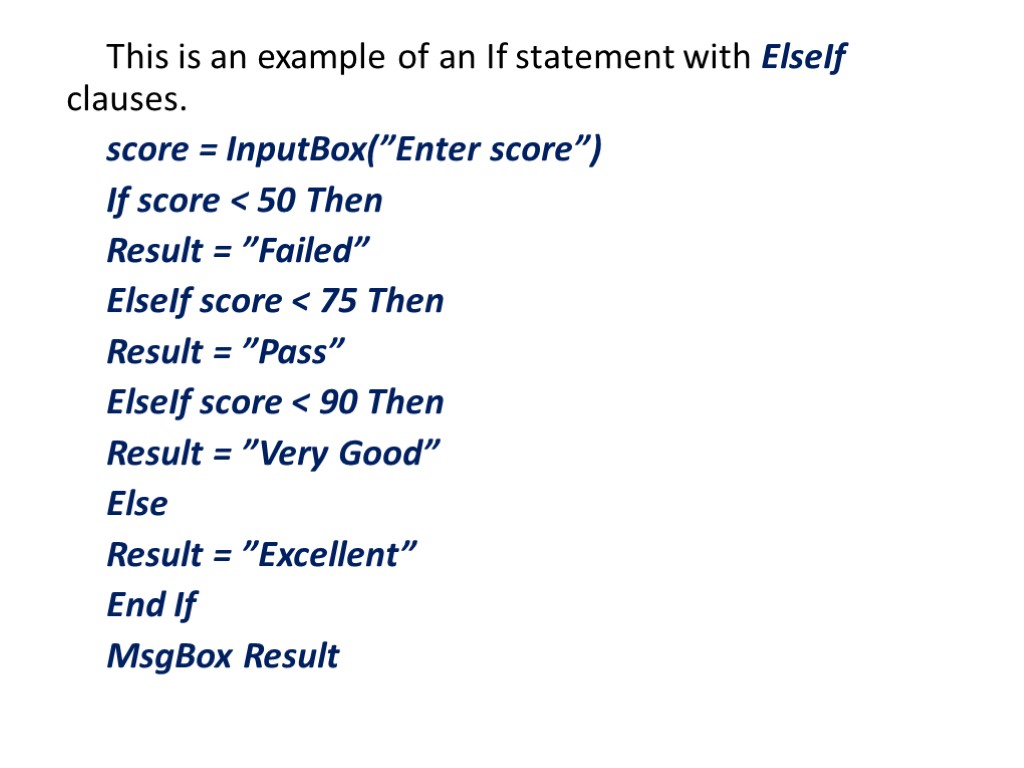
This is an example of an If statement with ElseIf clauses. score = InputBox(”Enter score”) If score < 50 Then Result = ”Failed” ElseIf score < 75 Then Result = ”Pass” ElseIf score < 90 Then Result = ”Very Good” Else Result = ”Excellent” End If MsgBox Result
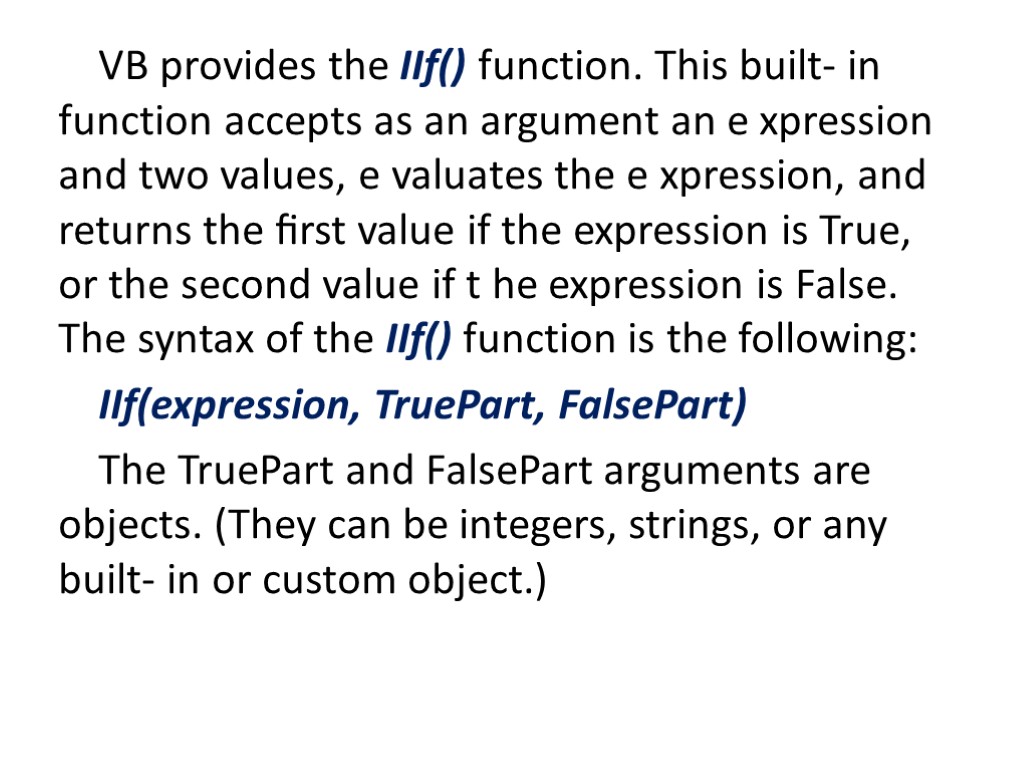
VB provides the IIf() function. This built- in function accepts as an argument an e xpression and two values, e valuates the e xpression, and returns the first value if the expression is True, or the second value if t he expression is False. The syntax of the IIf() function is the following: IIf(expression, TruePart, FalsePart) The TruePart and FalsePart arguments are objects. (They can be integers, strings, or any built- in or custom object.)
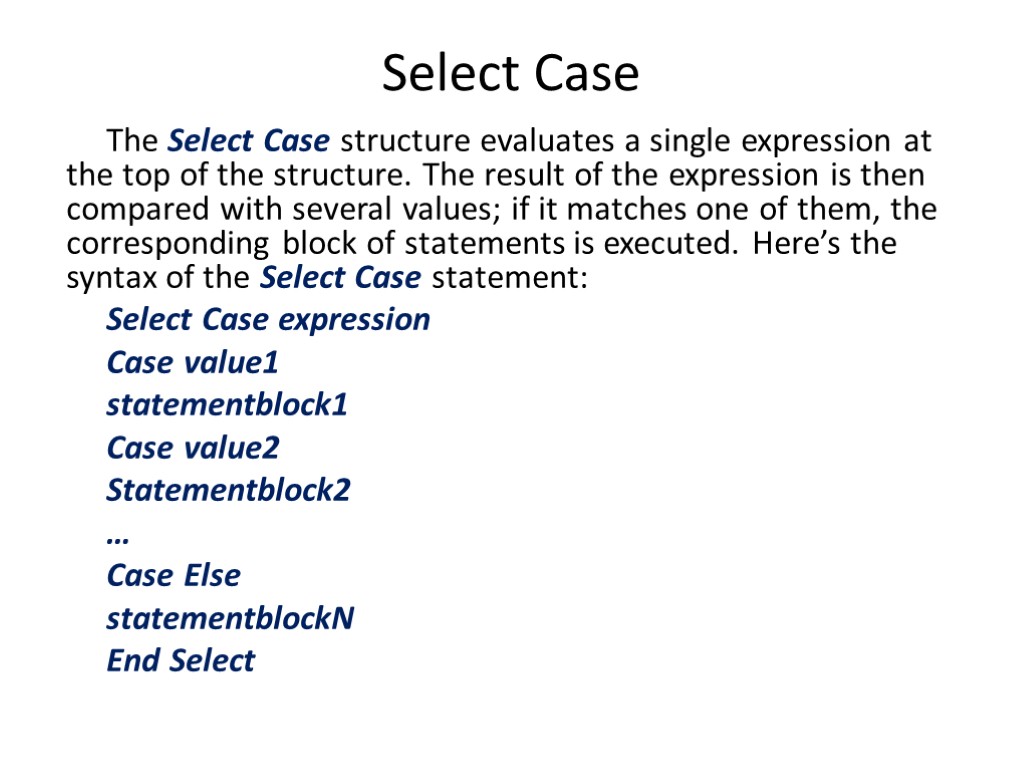
Select Case The Select Case structure evaluates a single expression at the top of the structure. The result of the expression is then compared with several values; if it matches one of them, the corresponding block of statements is executed. Here’s the syntax of the Select Case statement: Select Case expression Case value1 statementblock1 Case value2 Statementblock2 … Case Else statementblockN End Select
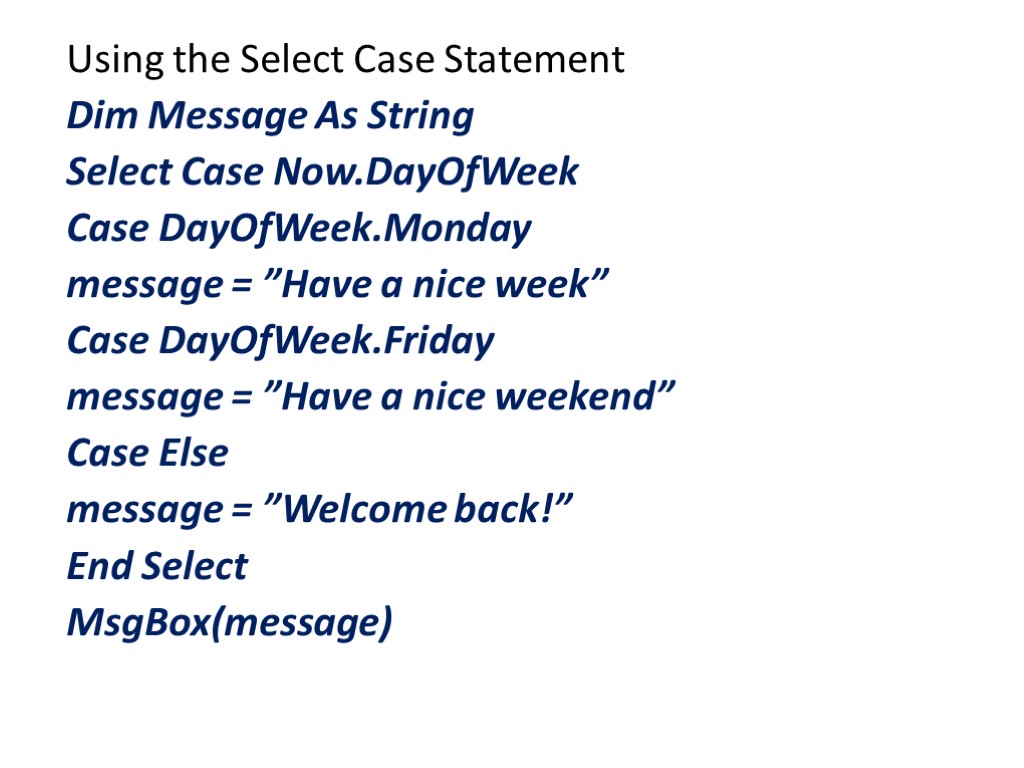
Using the Select Case Statement Dim Message As String Select Case Now.DayOfWeek Case DayOfWeek.Monday message = ”Have a nice week” Case DayOfWeek.Friday message = ”Have a nice weekend” Case Else message = ”Welcome back!” End Select MsgBox(message)
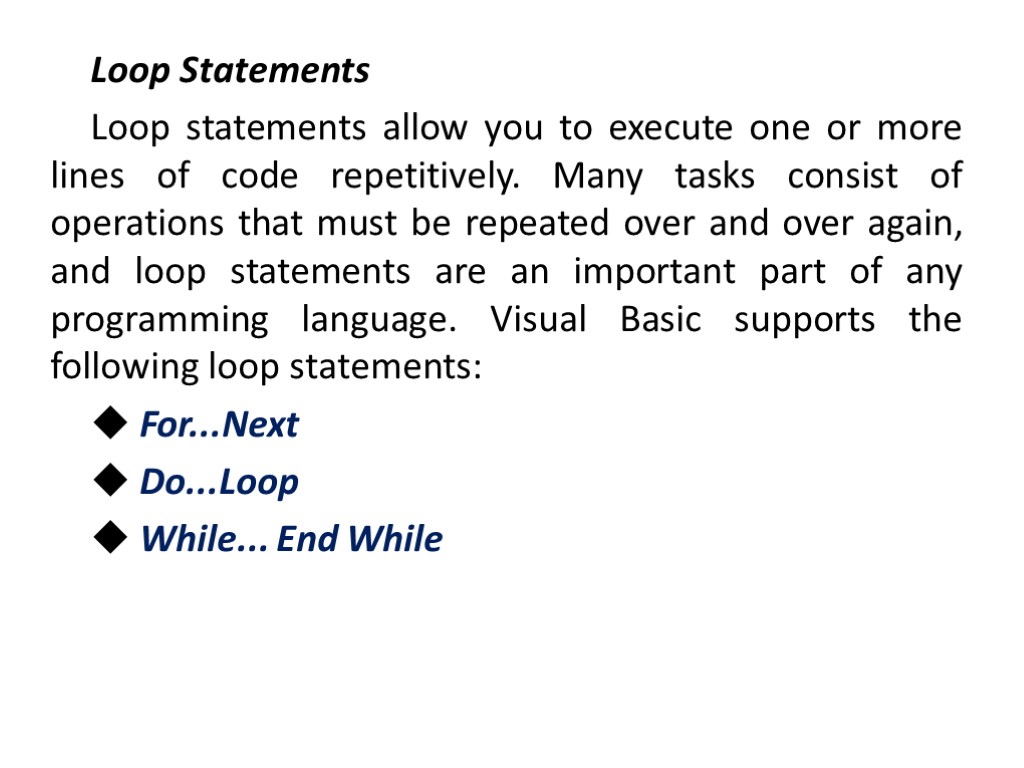
Loop Statements Loop statements allow you to execute one or more lines of code repetitively. Many tasks consist of operations that must be repeated over and over again, and loop statements are an important part of any programming language. Visual Basic supports the following loop statements: ◆ For...Next ◆ Do...Loop ◆ While... End While
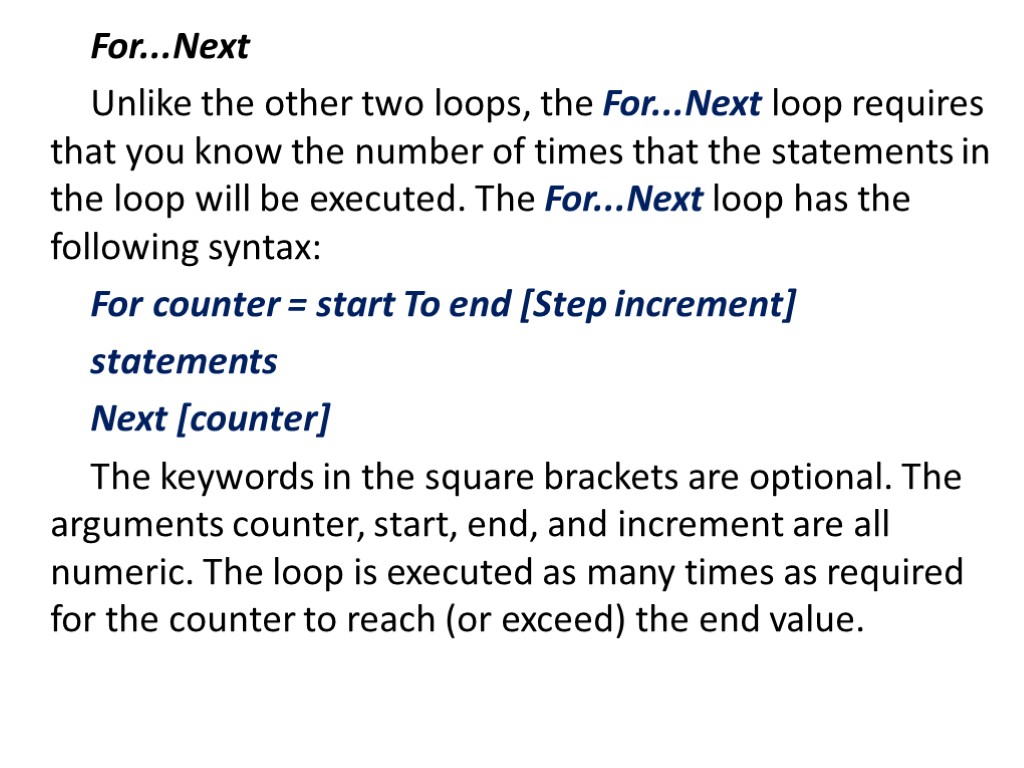
For...Next Unlike the other two loops, the For...Next loop requires that you know the number of times that the statements in the loop will be executed. The For...Next loop has the following syntax: For counter = start To end [Step increment] statements Next [counter] The keywords in the square brackets are optional. The arguments counter, start, end, and increment are all numeric. The loop is executed as many times as required for the counter to reach (or exceed) the end value.
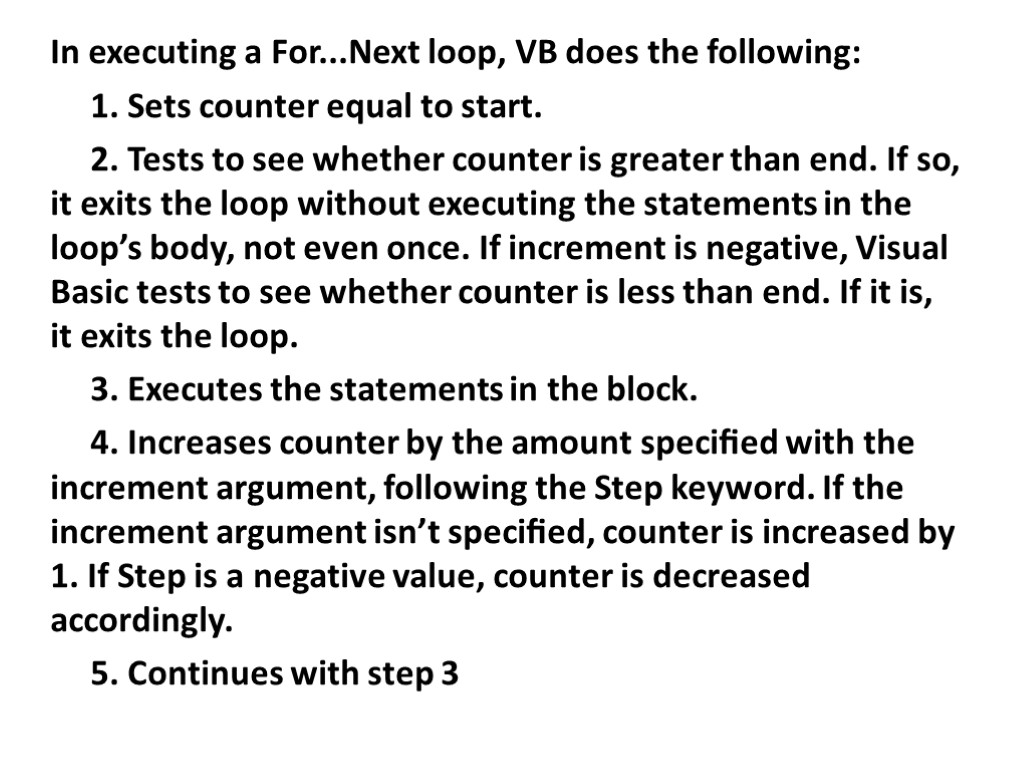
In executing a For...Next loop, VB does the following: 1. Sets counter equal to start. 2. Tests to see whether counter is greater than end. If so, it exits the loop without executing the statements in the loop’s body, not even once. If increment is negative, Visual Basic tests to see whether counter is less than end. If it is, it exits the loop. 3. Executes the statements in the block. 4. Increases counter by the amount specified with the increment argument, following the Step keyword. If the increment argument isn’t specified, counter is increased by 1. If Step is a negative value, counter is decreased accordingly. 5. Continues with step 3
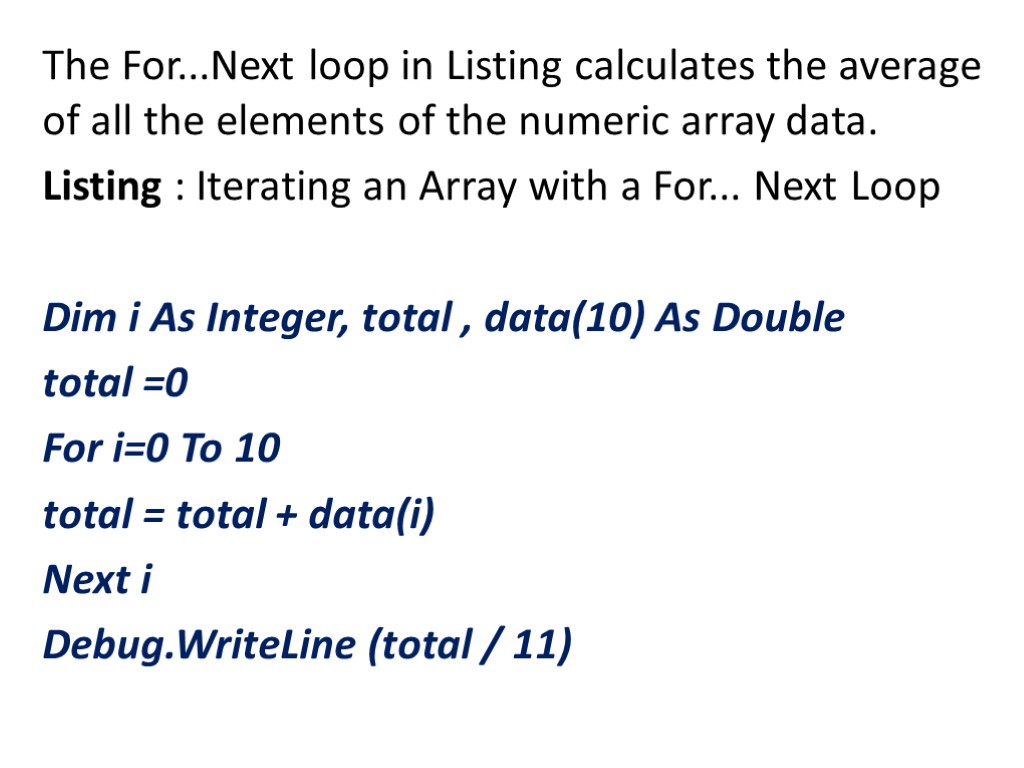
The For...Next loop in Listing calculates the average of all the elements of the numeric array data. Listing : Iterating an Array with a For... Next Loop Dim i As Integer, total , data(10) As Double total =0 For i=0 To 10 total = total + data(i) Next i Debug.WriteLine (total / 11)
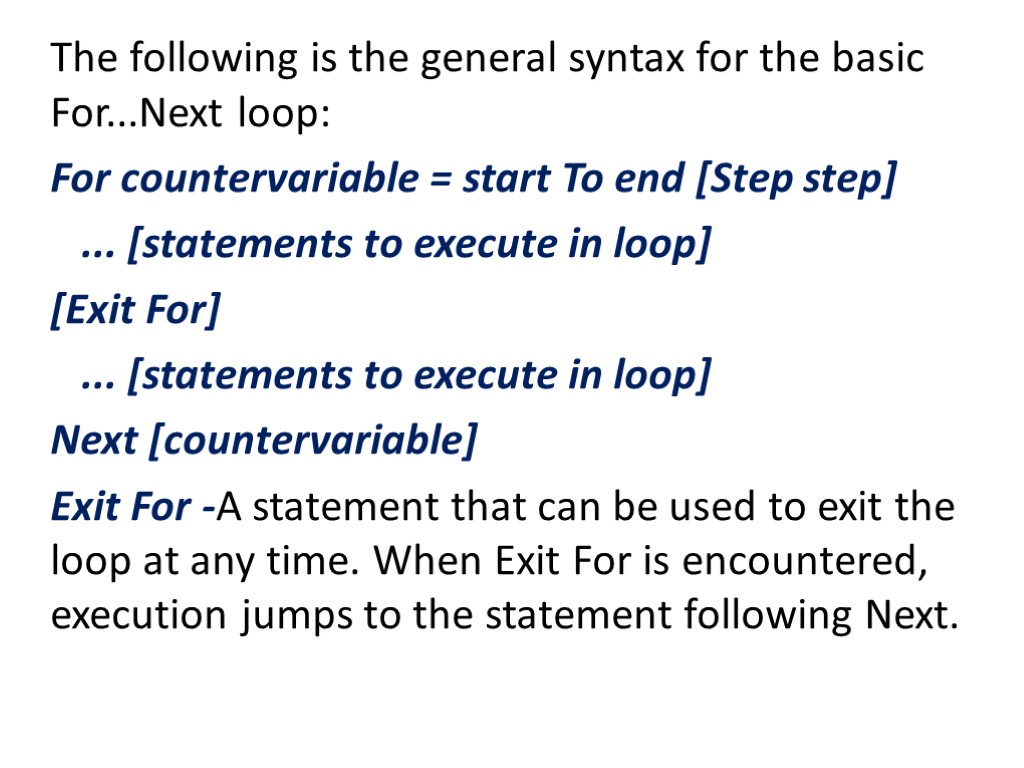
The following is the general syntax for the basic For...Next loop: For countervariable = start To end [Step step] ... [statements to execute in loop] [Exit For] ... [statements to execute in loop] Next [countervariable] Exit For -A statement that can be used to exit the loop at any time. When Exit For is encountered, execution jumps to the statement following Next.
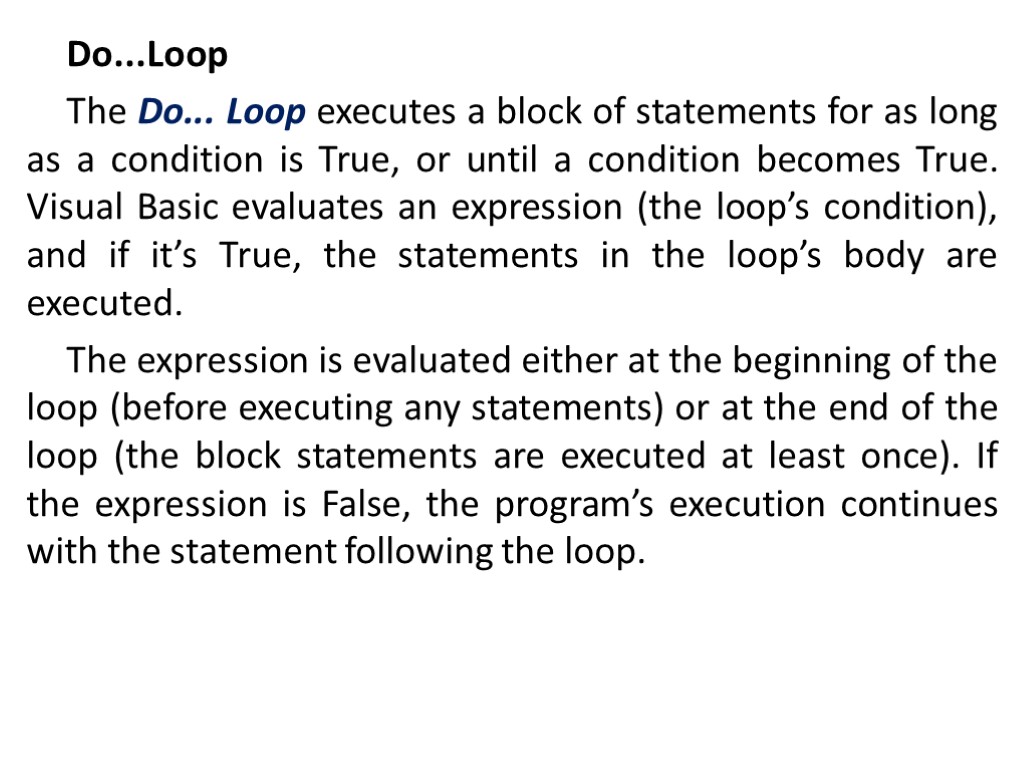
Do...Loop The Do... Loop executes a block of statements for as long as a condition is True, or until a condition becomes True. Visual Basic evaluates an expression (the loop’s condition), and if it’s True, the statements in the loop’s body are executed. The expression is evaluated either at the beginning of the loop (before executing any statements) or at the end of the loop (the block statements are executed at least once). If the expression is False, the program’s execution continues with the statement following the loop.
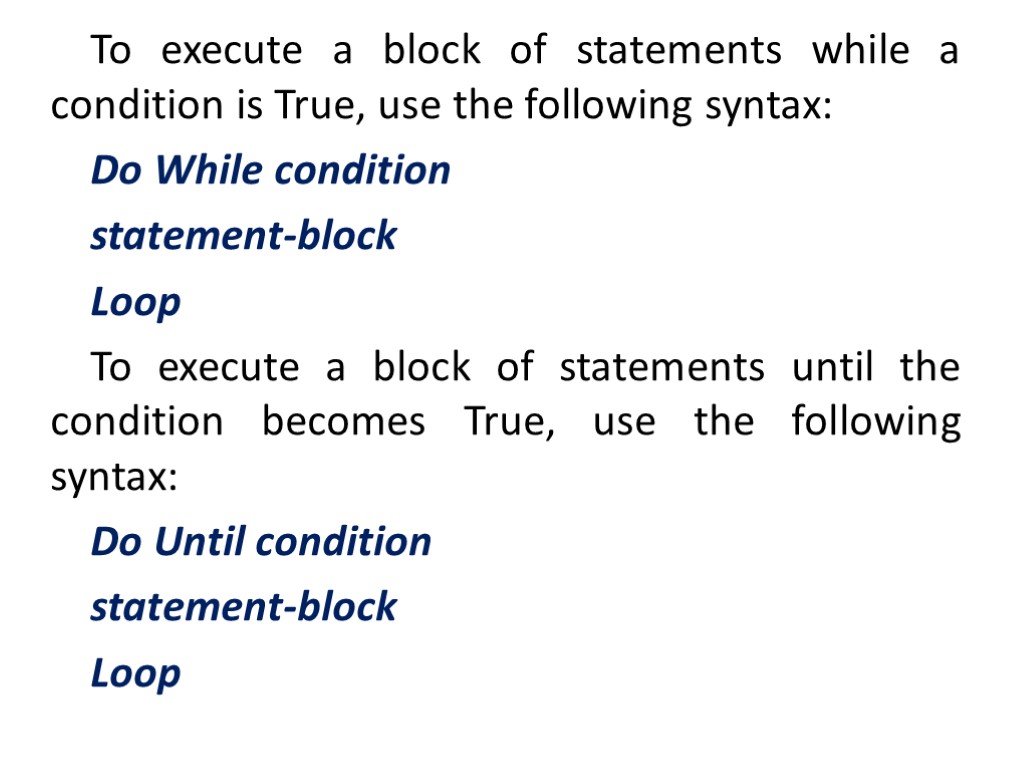
To execute a block of statements while a condition is True, use the following syntax: Do While condition statement-block Loop To execute a block of statements until the condition becomes True, use the following syntax: Do Until condition statement-block Loop
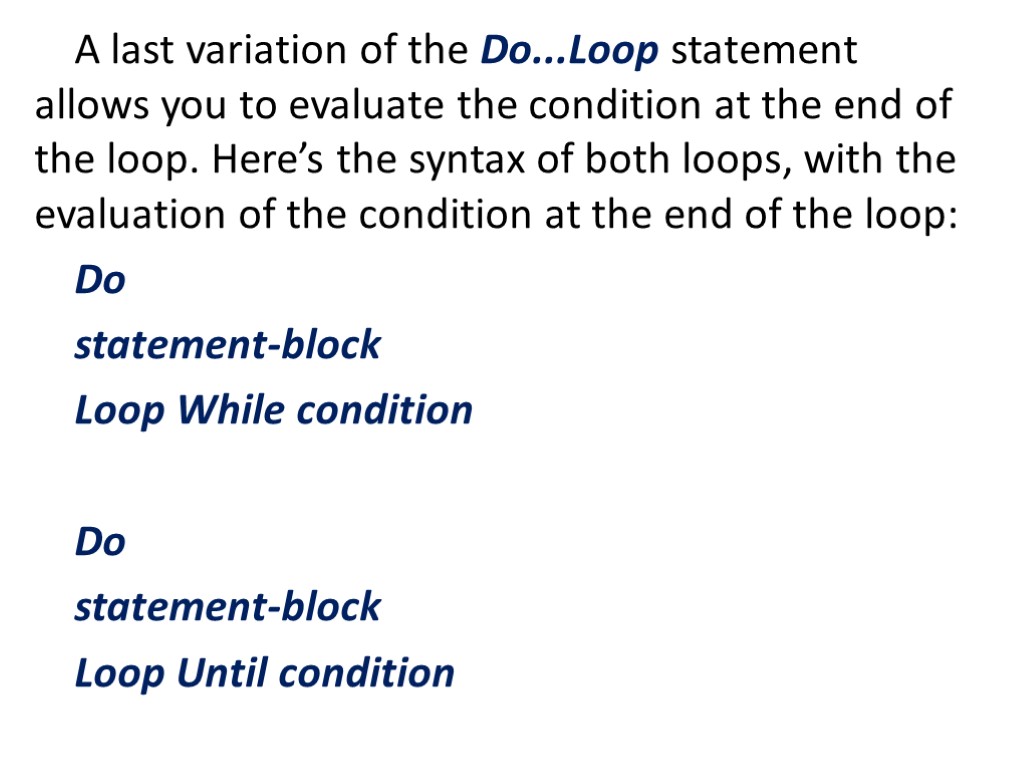
A last variation of the Do...Loop statement allows you to evaluate the condition at the end of the loop. Here’s the syntax of both loops, with the evaluation of the condition at the end of the loop: Do statement-block Loop While condition Do statement-block Loop Until condition
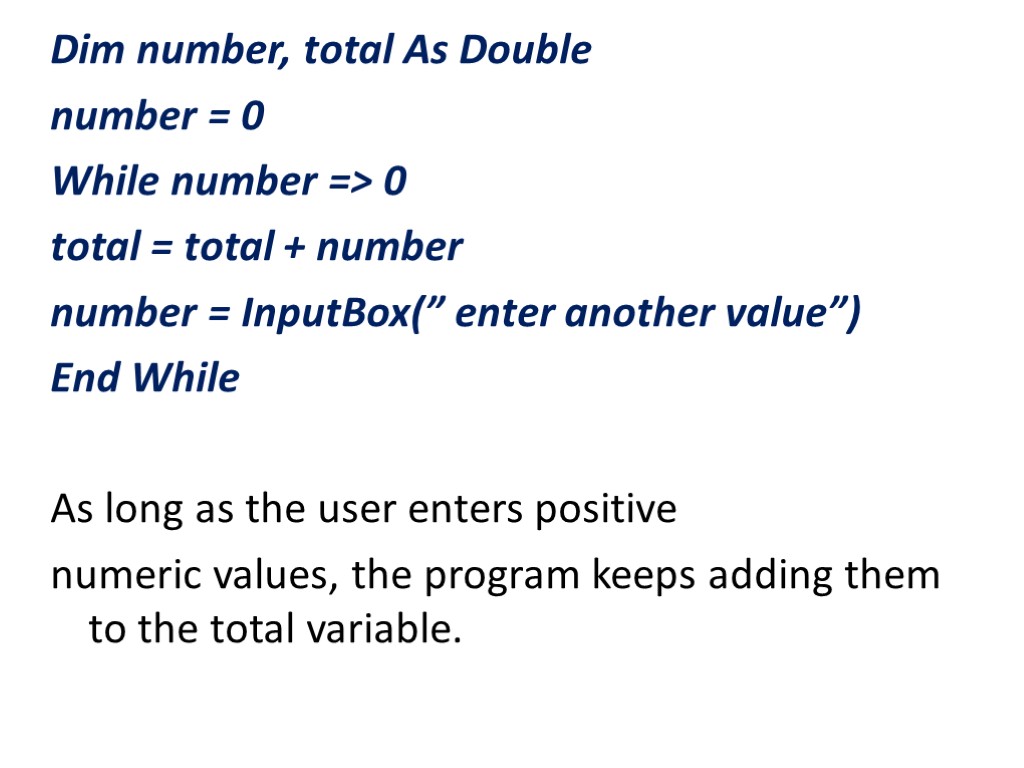
Dim number, total As Double number = 0 While number => 0 total = total + number number = InputBox(” enter another value”) End While As long as the user enters positive numeric values, the program keeps adding them to the total variable.

vb2.ppt
- Количество слайдов: 19